Tutorial: Execution Environment
In this tutorial we've been running code in the default environment and with the default Python interpreter. In a real project you may want to specify one or more of the following:
- Python interpreter and version
- PYTHONPATH
- Environment variables
- Initial run directory
- Options sent to Python
- Command line arguments
Wing lets you set these for your project as a whole and for specific files.
Project Properties
The Environment and Debug/Execute tabs in the Project Properties dialog, accessed from the Project menu, can be used to select the Python interpreter that is being used, the effective PYTHONPATH, the values of environment variables, the initial directory for the debug process, and any options passed to Python itself.
In most cases, Project Properties is where you will make changes to the runtime environment for all the project code that you execute and debug.
Try this out now by adding an environment variable TESTPROJECT=1 to Environment in Project Properties. Then restart debugging and look at os.environ in the Debug Console to confirm that the new environment variable is defined.
File Properties and Launch Configurations
File Properties are used to configure the command line arguments sent to a file when it is executed or debugged, and optionally to override the project-defined environment on a file by file basis.
The File Properties dialog is accessed from the Current File Properties item in the Source menu or by right-clicking on a file in the editor or Project tool and selecting Properties.
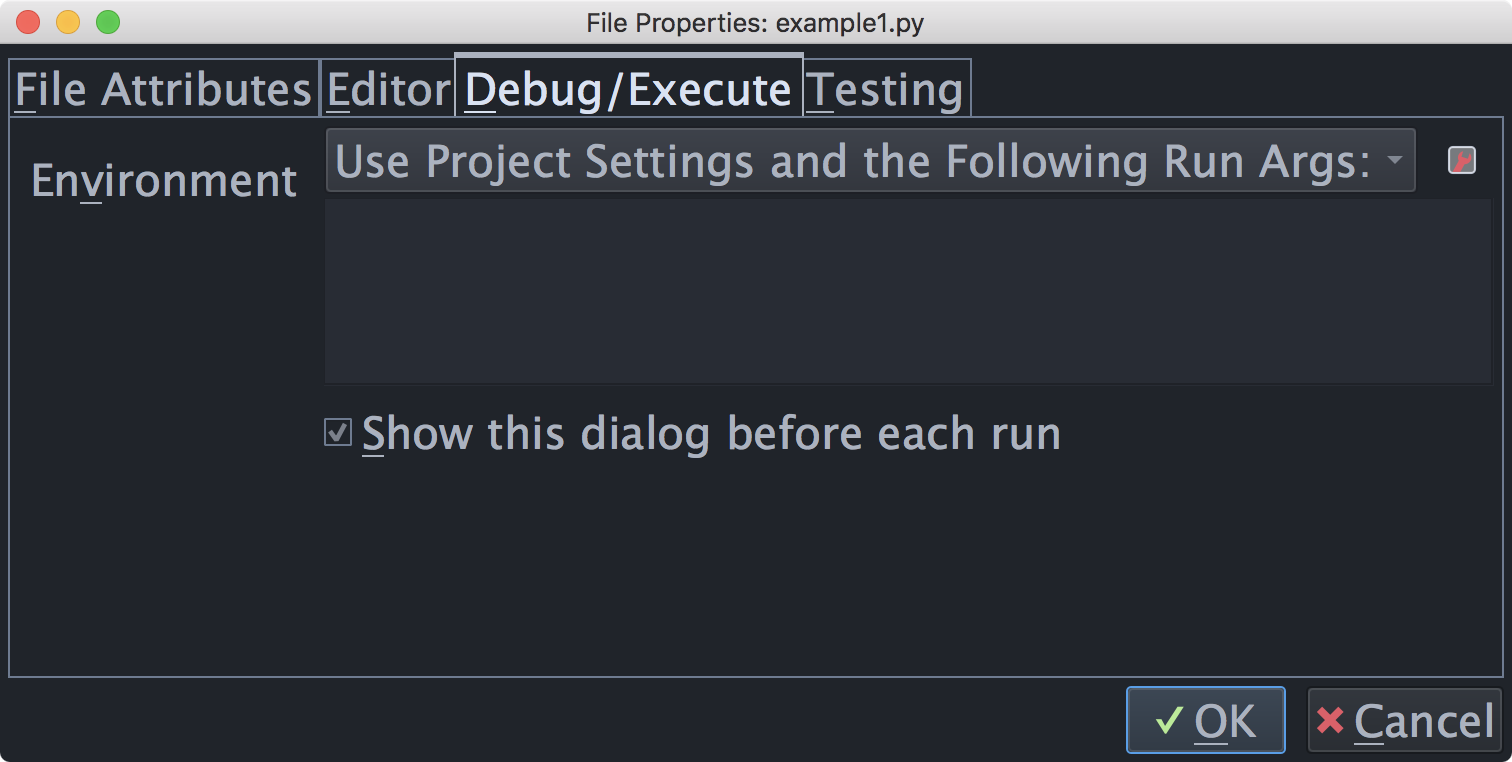
The most common use of File Properties is simply to set the command line arguments to use with the file. Try this now by bringing up File Properties for example1.py and set the run arguments in the Debug/Execute tab to test args.
Now if you restart debugging and type the following in the Debug Console you will see that the environment and arguments have been set:
os.environ.get('TESTPROJECT')
sys.argv[1:]
The output should be:
1 ['test', 'args']
To also override the project-defined environment for a particular file, define a Launch Configuration and select it in File Properties. This sets up an environment like that which can be specified in Project Properties and pairs it with a particular set of command line arguments. A launch configuration can be reused with multiple files or in Named Entry Points (see below).
Try this now by bringing up File Properties for example1.py again and selecting Use Selected Launch Configuration for Environment under the Debug/Execute tab. Press the New button that appears, use``My Launch Config`` as the name for the new launch configuration, and press OK. Wing will show the properties dialog for the new launch configuration.
Next enter run arguments other args and change the Environment to Add to Project Values and enter TESTFILE=2 and TESTPROJECT=. This adds environment variable TESTFILE and removes the TESTPROJECT from the inherited project-defined environment.
Now restart debugging again and enter this in the Debug Console:
os.environ.get('TESTPROJECT')
os.environ.get('TESTFILE')
sys.argv[1:]
The output should be:
None 2 ['other', 'args']
See File Properties and Launch Configurations for details.
Main Entry Point
You can specify one file in your project as the main entry point for debugging and execution. When this is set, debugging will always start there unless you use Debug Current File in the Debug menu.
To set a main entry point use Set Current as Main Entry Point in the Debug menu, right click on the Project tool and select Set as Main Entry Point, or use the Main Entry Point property under the Debug tab of the Project Properties dialog.
Try this now by setting example1.py as the main entry point. After doing so, it is no longer necessary to bring example1.py to front in order to start debugging it.
Whether or not you set a main entry point depends on the nature of your project.
See Specifying Main Entry Points for details.
Named Entry Points
In some projects it is more convenient to define multiple entry points for executing and debugging code. To accomplish this, Named Entry Points can be set up from the Debug menu. Each named entry point binds an environment, either specified in the project or in a launch configuration, to a particular file. Once defined, they can be assigned a key binding or accessed from the Debug Named Entry Point and Execute Named Entry Point items in the Debug menu.
Named Entry Points are a good way to launch a single file with different arguments or environment.
See Named Entry Points for details.